If you are looking to stream your content along with DRM, chances are that you’re bound to come across Shaka player. As an open-source JS library, the Shaka player is widely used for adaptive video streaming. You can play content based on DASH and HLS, without browser plugins, with the help of an Encrypted media extension. So, in order to help others out and make it easier for others to learn about using Shaka player for DRM playback, I wrote this article.
So, without further ado, let’s get started.
What is Encrypted Media Extension (EME)
DRM playback is based on the Encrypted Media Extension Technology, which is the standard of W3C to provide DRM playback support in web browsers.
EME can be majorly divided into two parts:
EME API:
These APIs are the part of the browser API as the standard of W3C to securely communicate between the Video Element and CDM (Content Decryption Module) where actual decoding of media happens
Content Decryption Module:
CDM is a proprietary software/hardware of DRM service provider that can be bundled in the browser/OS or added as hardware in the client machine, it’s the main purpose is to decode the video in a secure executing environment without exposing it to the user and rendering media directly on the video element.
Here’s a brief block diagram of EME Tech in Vdophiper Player and Playback.
Both DRM service providers, Apple Fairplay DRM and Google Widevine DRM provide CDM in Safari and Chrome browsers respectively.
For video playback, Apple expects developers to consume EME API and provide playback on the video element, but google Widevine goes one step ahead and also provides JS video Player name Shaka Player that provides full basic playback using EME API and Video Element.
About Vdocipher Player:
Vdocipher Player is a plug-n -play video player, that expects the VideoID and OTP and handles everything under the hood, like choosing the DRM service based on the user device and provide basic playback,
Also, this player is loaded with tons of features like playlist, video analytics, captions and many more.
Explore More ✅
VdoCipher empowers course creators, event organizers and broadcasters with expert live video streaming, ensuring smooth playback globally.
What is Shaka Player?
Shaka Player is an open-source js video player mainly used for DRM service maintained by google. Under the hood for DRM service, it actuals bind the Video Element and Widevine CDM by consuming the EME API
Features:
- Supports the Adaptive Bitrate Streaming, which means the quality of media adapts to the bandwidth of the user.
- Can also support offline storage and playback using IndexDB
- Also, provide option UI Lib for player
- Easy integration with Chromecast and also supports the Apple Fairplay.
- Open-source
Steps to implement Shaka Player for Widevine
- Setup the basic video player
- Add the DRM configuration
- Handle License Server Authentication & delivers licenses
Setup The Basic video player
In this setup, we will add the video without DRM using shaka player lib.
<html> <head> <!-- Shaka Player compiled library: --> <script src="dist/shaka-player.compiled.js"></script> </head> <body> <video id="video" width="640" poster="example.com/poster.jpg" controls autoplay ></video> </body> <script> // Video Manifest URL const manifestUri = "https://storage.googleapis.com/shaka-demo-assets/angel-one/dash.mpd"; // Install all the required Polyfill shaka.polyfill.installAll(); if (shaka.Player.isBrowserSupported()){ throw new Error("Browser not supported !") }; const video = document.getElementById("video"); //Shaka Player Reference provides all methods and properties. const player = new shaka.Player(video); player.addEventListener("error", onErrorEvent); player .load(manifestUri) .then(() => console.log("Video Load successful")) .catch((error) => console.error("Error code", error.code, "object", error) ); </script> </html>
Add the DRM configuration.
For DRM playback we need to add the DRM configuration to the player instance we created above ( in between the script tags )
player.configure({ DRM: { servers: { 'com.widevine.alpha': 'https://YOUR.AUTHENTICATION.SERVER', } } })
Shaka player will request https://your.authentication.server/,to get the License Certificate,
Shaka Player is a key-system-agnostic, it uses EME to ask the browser what its key-system is supported, and make no assumptions. If your browser supports multiple key systems, the first supported key system in the manifest is used
Handling License Server Authentication
Your application’s license server may require some form of authentication so that it only delivers licenses to paying users,
but for that you need to add header/token/parameter with each request to identify the user, you can do so by interrupting the request for License Certificate, and send them the License Certificate only if it passed the authorization criteria.
To interrupt the License Certificate request we will use the `getNetworkEngine` Method
player .getNetworkingEngine() .registerRequestFilter(function (type, request) { // Only add headers to license requests: if (type == shaka.net.NetworkingEngine.RequestType.LICENSE) { // This is the specific header name and value the server wants: request.headers["user-identify-token"] = "VGhpc0lzQVRlc3QK"; } });
In the above, we add the parameter in the request header, although there are two more options like Cookie Authentication, Parameter Authentication. To identify the right user on License Server.
Steps to implement Shaka Player for FairPlay
Shaka players also support Apple FairPlay DRM, Implementing the FairPlay the follow same above three-step paradigm as implementing the Widevine.
First step remains the same in both, it just the DRM configuration and handling the request changes while implementing the FairPlay
player.configure({ DRM: { servers: { "com.apple.fps.1_0": licenseUri, }, advanced: { "com.apple.fps.1_0": { serverCertificate: fairplayCert, }, }, initDataTransform: function (initData) { const skdUri = shaka.util.StringUtils.fromBytesAutoDetect(initData); console.log("skdUri : " + skdUri); const contentId = skdUri.substring(skdUri.indexOf("skd://") + 6); console.log("contentId : ", contentId); const cert = player.drmInfo().serverCertificate; return shaka.util.FairPlayUtils.initDataTransform( initData, contentId, cert ); }, }, }); player.getNetworkingEngine().registerRequestFilter(function (type, request) { if (type == shaka.net.NetworkingEngine.RequestType.LICENSE) { const originalPayload = new Uint8Array(request.body); const base64Payload = shaka.util.Uint8ArrayUtils.toBase64(originalPayload); const params = "spc=" + encodeURIComponent(base64Payload); request.body = shaka.util.StringUtils.toUTF8(params); request.headers["user-identify-token"] = authToken; // Token to identify the user. } }); player.getNetworkingEngine().registerResponseFilter(function (type, response) { // Alias some utilities provided by the library. if (type == shaka.net.NetworkingEngine.RequestType.LICENSE) { const responseText = shaka.util.StringUtils.fromUTF8(response.data).trim(); response.data = shaka.util.Uint8ArrayUtils.fromBase64(responseText).buffer; parsingResponse(response); } });
Shaka Player UI
Shaka player also ships the UI controls for the video element, to implement UI, use the shaka-player.ui.js build and add the attribute of data-shaka-player-container of parent div of the video element. It will add the controls in that div.
<div data-shaka-player-container> <video autoplay data-shaka-player id="video"></video> </div>
And to access the Player instance and Controls instance
const video = document.getElementById("video"); const ui = video["ui"]; const controls = ui.getControls(); const player = controls.getPlayer(); player.addEventListener("error", console.error); controls.addEventListener("error", console.error);
To configure the UI of Player we can use UI. configure(configure) method.
const config = { addSeekBar: false, controlPanelElements: ["rewind", "fast_forward"], seekBarColors: { base: "rgba(255, 255, 255, 0.3)", buffered: "rgba(255, 255, 255, 0.54)", played: "rgb(255, 255, 255)", }, }; ui.configure(config);
Offline Storage and Playback
Shaka player also provides the offline playback of video with DRM solution, it uses the index DB to store the video and Service worker to map the license request.
Conclusion
I hope this article helped you on how to ensure Shaka Player implementation for DRM playback. In this article, I’ve gone through what Shaka player and EME are. Also, how you can set up the player, add DRM configuration, and more.
At Vdocipher, we implement Shaka player for DRM playback on our own. When you use our platform, you won’t have to worry about implementing Shaka player or DRM. We take care of all of it, all you have to do is simply upload your video to our platform and embed it on your website.
Supercharge Your Business with Videos
At VdoCipher we maintain the strongest content protection for videos. We also deliver the best viewer experience with brand friendly customisations. We'd love to hear from you, and help boost your video streaming business.
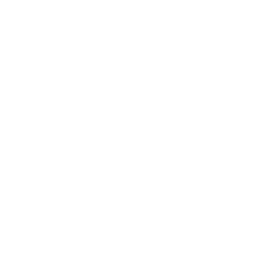